Using ALPON X4 as a Security Camera
A Python-based project for ALPON X4 that detects humans via a security camera, sends notifications using ntfy.sh, and operates within a Docker container.
This guide explains how to set up a Python application on the ALPON X4 device to detect humans using a security camera. When a human is detected or no longer visible, notifications are sent via ntfy.sh. The application uses OpenCV and the Haar-cascade classifier and runs within a Docker container, uploaded to the Sixfab Registry. Below are the detailed instructions for setup, deployment, and customization.
Project Overview
This project detects human presence in real time using a security camera and sends notifications when a human is detected. The notifications are delivered through ntfy.sh:
- Human detected: A notification “Human detected!” will be sent when a person is visible in front of the camera.
Camera Support
A USB camera is assumed for the default setup. However, the code can be modified to support other camera protocols, such as RTSP. OpenCV, which powers this application, supports a wide range of camera inputs. ALPON X4 imposes no restrictions in this regard. For more details on supported cameras, refer to the OpenCV Camera Documentation.
USP Port
Ensure that the camera is connected to the upper USB port of the ALPON X4. The application is configured to work with this port, as indicated by the device’s USB port mapping.
Pre-Deployment System Preparation
Before deploying the application, follow these steps to prepare your system:
-
Update the System
Update your ALPON X4 to the latest software version:
sudo apt update && sudo apt upgrade -y
-
Install Video4Linux Utilities
Install the necessary tools for USB camera support:
sudo apt install v4l-utils -y
-
Check Camera Detection
Use the following command to list available cameras:
v4l2-ctl --list-devices
Example output:
Source Code
Below is the Python code that runs inside a Docker container.
import cv2
import requests
# Load the Haar-cascade model
haar_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml')
# Open the video source
cap = cv2.VideoCapture(0)
# ntfy.sh settings
NTFY_URL = "https://ntfy.sh/YOUR_TOPIC_NAME"
HUMAN_DETECTED_MSG = "Human detected!"
CAMERA_ERROR_MSG = "Camera error."
# Check if the camera opened successfully
if not cap.isOpened():
requests.post(NTFY_URL, data=CAMERA_ERROR_MSG)
print(CAMERA_ERROR_MSG)
exit(1) # Exit the script if the camera cannot be opened
while True:
ret, frame = cap.read()
if not ret:
break
# Convert to grayscale for detection
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
faces_rect = haar_cascade.detectMultiScale(gray, 1.1, 9)
if len(faces_rect) > 0:
requests.post(NTFY_URL, data=HUMAN_DETECTED_MSG)
print(HUMAN_DETECTED_MSG)
cap.release()
Subscribing to Notifications
Change the NTFY_URL variable to use your own ntfy.sh topic. Open the application code and locate the following line:
Replace YOUR_TOPIC_NAME
with your chosen topic name. For example:
Copy the URL from the code and open it in your web browser. This will automatically subscribe you to the topic.
Setup Instructions
1. Download Haar-cascade Model
Download the haarcascade_frontalface_default.xml file from the OpenCV GitHub repository.
- Open haarcascade_frontalface_default.xml file from the OpenCV repository.
- Click the download raw file button.
- Move the model file to your project directory.
2. Create a Docker Container
Use the following Dockerfile to package your application:
# Base image
FROM python:3.9-slim
RUN apt-get update && apt-get install -y \
libgl1-mesa-glx \
libglib2.0-0 \
libsm6 \
libxrender1 \
libxext6
# Copy project files into the container
COPY . /app
WORKDIR /app
# Install required Python libraries
RUN pip install opencv-python requests
# Run the application
CMD ["python3", "main.py"]
3. Build and Upload Docker Container
- Build the Docker container using the following command:
docker buildx build --platform linux/arm64 -t security_cam:latest ./
- Upload the container to Sixfab Registry:
- Navigate to the Sixfab Registry page.
- Click on "+ Add Container" and follow the prompts.
4. Deployment
- Navigate to the Applications tab of your asset and click "+ Deploy".
- Deploy the app using the following settings:
- Enter container name.
- Select the image and tag you uploaded to the Sixfab Registry.
- Enable the "Privileged" section for camera access.
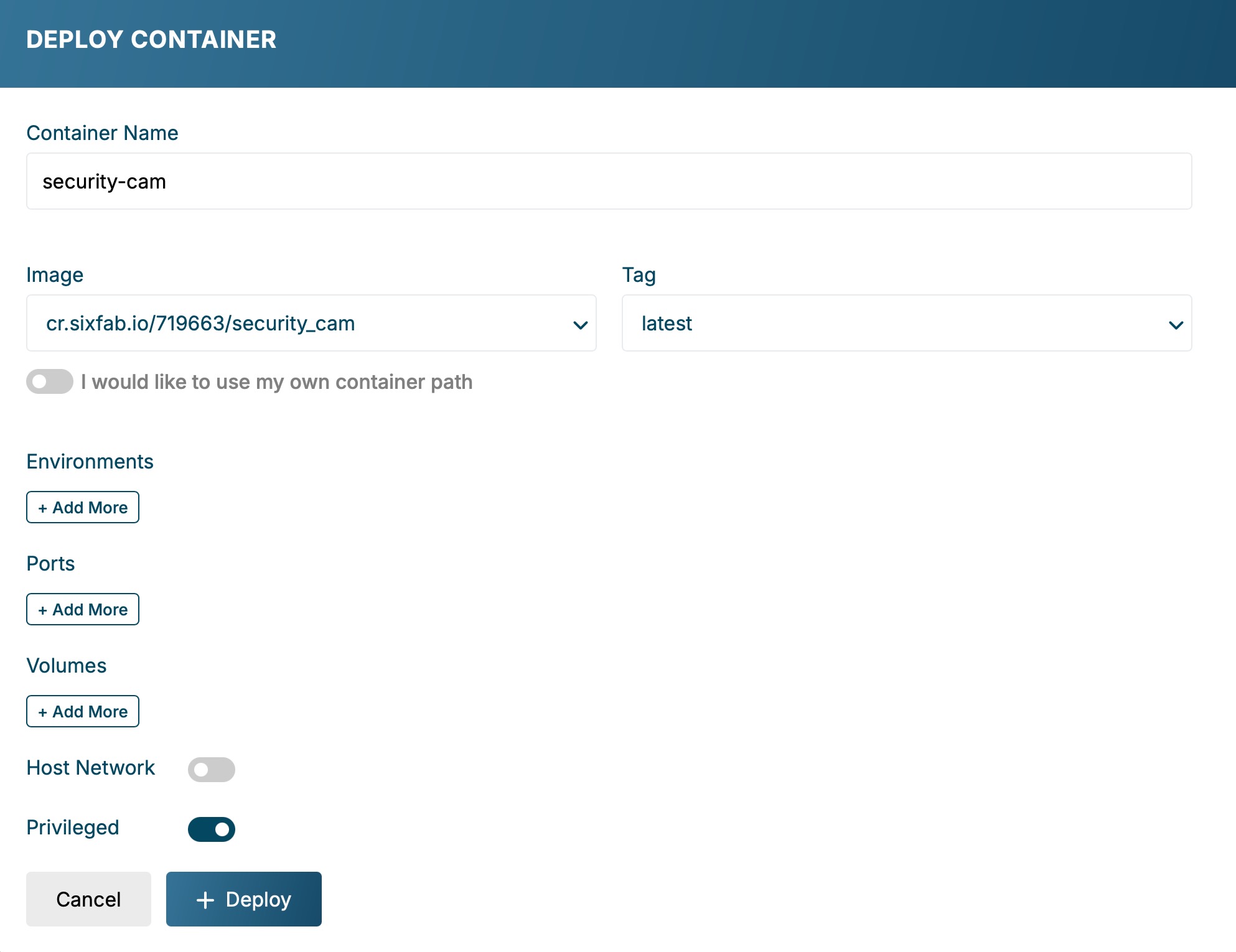
5. Notification Sent Successfully
After successfully deploying the app, notifications will now be delivered to this topic when a human is detected.
Below is a screenshot showing that the "Human detected!" notification was successfully sent via ntfy.sh. This confirms that the application is working correctly and notifications are being sent to the ntfy.sh service.
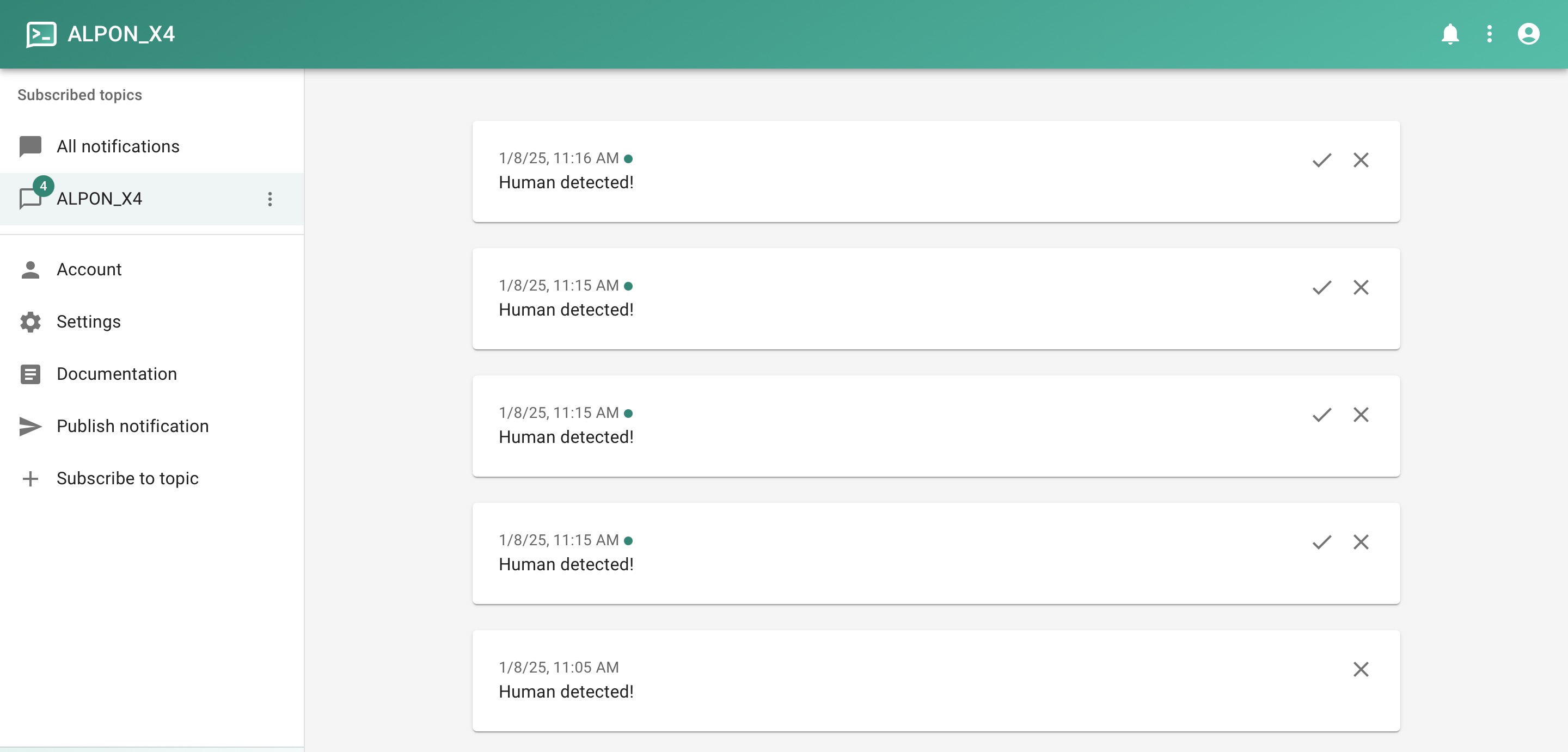
Customizing Notifications Messages
You can modify the notification messages that will be sent by changing the following variables in the code:
HUMAN_DETECTED_MSG - The message sent when a human is detected.
Troubleshooting
- Camera Access Issues: If the camera does not open, ensure that the camera drivers are installed on the ALPON X4.
- Haar-cascade Model Not Found: Ensure that the haarcascade_frontalface_default.xml file is in the correct location.
- ntfy.sh Notifications Not Sent: Check your internet connection and ensure the ntfy.sh URL is correct.
Updated 4 months ago