Troubleshooting
This guide aims to empower users with the knowledge and tools required to identify, diagnose, and resolve technical issues related to Sixfab Pico LTE
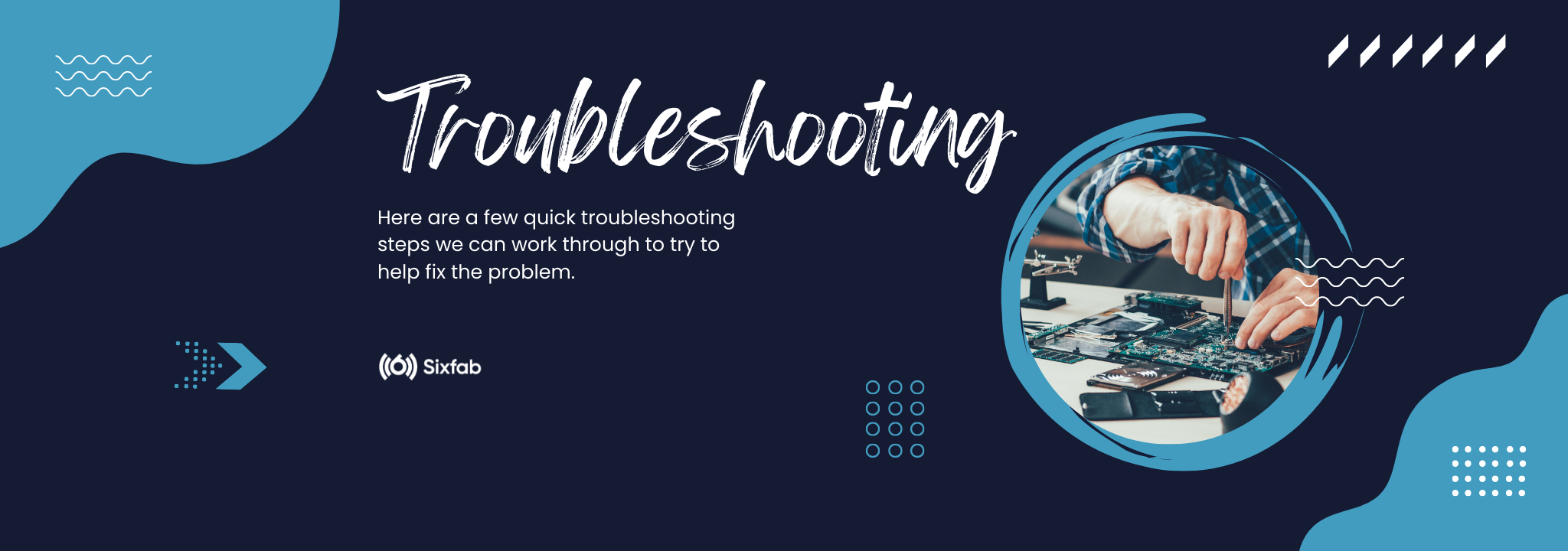
This comprehensive resource has been created to assist you in diagnosing and resolving technical issues you may encounter while utilizing the Pico LTE SDK in your MicroPython projects.
Enabling Debug Mode
By enabling the debug mode on the Pico LTE SDK, you can access detailed output that will help you pinpoint and troubleshoot problems effectively. To enable debug mode in the Pico LTE MicroPython SDK, follow these steps:
- Import the debug class with the following code:
from pico_lte.common import debug
- Then enable it by setting the debug level:
from pico_lte.common import debug
debug.set_level(0)
That's it! With these two lines of code, debugging mode will be enabled for your Pico LTE project. Once debug mode is enabled, running your Pico LTE project will generate additional output that can be used for troubleshooting purposes.
AT Commands
By sending AT commands to the Pico LTE module, you can gather a wealth of information and effectively troubleshoot issues with your Pico LTE.
Here's an example of how you can send an AT command to the Pico LTE:
from pico_lte.utils.atcom import ATCom
# Instantiate the ATCom object
atcom = ATCom()
# Send the AT command to the Pico LTE
at_command = "YOUR_AT_COMMAND"
response = atcom.send_at_comm(at_command)
# Display the response received from the LTE module
print(response)
To send an actual AT command, replace "AT_COMMAND" with the specific AT command you want to send. For example, if you want to retrieve the signal strength, you can use the command "AT+CSQ".
If you need to include the character " within an AT command, you should define the string between single quotation marks. For example, consider the following line:
Also, you can use a backslash ( \ ) before the quotation mark in the string. For example:
The function send_at_comm() has six parameters. The parameter "command" is compulsory to use, while the others are optional and can be used as needed.
command: str
desired: str or list, default: None
fault: str or list, default: None
timeout: int, default: 5
line_end: bool, default: True
urc: bool, default: False
- The “command” parameter holds AT command to be sent. It is a positional parameter and can be used without specifying parameter name. For example: send_at_comm("AT")
- The "desired" and "fault" parameters are used to capture one or more phrases in the response. If the "desired" parameter is found in the response, the function returns "SUCCESS." Similarly, if the "fault" parameter is found, "ERROR" is returned. For example: send_at_comm("AT+CREG?", desired=["+CREG: 0,1", "+CREG: 0,5"], fault="+CREG: 0,2")
- The "timeout" parameter holds the timeout for receiving a response in seconds.
- If the "line_end" parameter is set to "True" (boolean), the function appends a line-ending character ('\r') to the end of the "command.”
- The "urc" parameter, when set to "True" (boolean), is used to receive Unsolicited Result Codes (URC) responses. URCs are messages that are not the result of an AT command. This parameter is necessary for some of the AT commands, such as "AT+QHTTPPOST," "AT+QHTTPGET," "AT+QHTTPPUT," "AT+QHTTPREAD," and
"AT+QHTTPURL." It is also required for sending specific data, such as request headers and request bodies, without using AT Commands.
Once you have specified the desired AT command, execute the code. The send_at_comm method of the ATCom object will send the AT command to the Pico LTE, and the response from the module will be stored in the response variable.
Finally, the print statement will display the response received from the LTE module, allowing you to view and analyze the output.
If you receive {'response': 'timeout', 'status': 2}, it means your LTE module is powered off. Press the PWRKEY button to turn on the module. The Status LED will start blinking.
We will explore some useful AT commands that can be sent to the Pico LTE to enhance functionality and facilitate efficient communication.
AT+CMEE | Enable or Disable Error Reporting |
---|---|
Meaning | This command enables or disables extended error reporting. When enabled, it provides detailed error codes for better troubleshooting. |
Example | AT+CMEE=1 to enable extended error reporting. |
AT+CREG | Network Registration Status |
---|---|
Meaning | This command retrieves the current network registration status of the module. |
Example | AT+CREG? to query the network registration status. |
AT+CSQ | Signal Quality |
---|---|
Meaning | This command retrieves the signal quality and received signal strength indication (RSSI) values. |
Example | AT+CSQ to retrieve the signal quality. |
AT+QNWINFO | Network Information |
---|---|
Meaning | This command retrieves information about the currently registered network, such as the MCC (Mobile Country Code) and MNC (Mobile Network Code). |
Example | AT+QNWINFO to retrieve network information. |
AT+CGACT | PDP Context Activation |
---|---|
Meaning | This command activates or deactivates a PDP (Packet Data Protocol) context for data transmission. |
Example | AT+CGACT=1,1 to activate a PDP context. |
AT+CGATT | Attach or Detach from GPRS Service |
---|---|
Meaning | This command is used to attach or detach the module from the GPRS (General Packet Radio Service) network. |
Example | AT+CGATT=1 to attach to the GPRS service. |
AT+COPS | Operator Selection |
---|---|
Meaning | This command is used to manually select or automatically search for an operator to register the module on a specific network. |
Example | AT+COPS=0 to automatically select an operator. |
AT+CGPADDR | PDP Address |
---|---|
Meaning | This command retrieves the IP address assigned to the active PDP context. |
Example | AT+CGPADDR=1 to retrieve the IP address for PDP context 1. |
Please check the module documentation for more AT command details.
By leveraging the power of AT commands, you can gather vital information from the module and gain deeper insights into the issues you are facing. This will significantly contribute to resolving problems and optimizing your Pico LTE projects.
Connection Time Too Long
The BG95-M3 modem supports eMTC (LTE CAT-M1) and GSM networks; however, NB-IoT is disabled. By default, the modem scans networks in the following order: eMTC, NB-IOT, and GSM. Therefore, if eMTC is not available in your region, the modem will attempt to connect to a GSM network. In some cases, this operation may take a long
time. To reduce connection time, you can manually change the scan sequence as below.
from pico_lte.utils.atcom import ATCom
from pico_lte.modules.base import Base
# Instantiate the ATCom object
atcom = ATCom()
# Instantiate the Base object
base = Base(atcom)
"""
Set scan sequence (default=00)
* 00 --> Automatic (eMTC → NB-IoT → GSM)
* 01 --> GSM priority
* 02 --> eMTC priority
"""
response = base.config_network_scan_sequence("01")
# Display the response received from the LTE module
print(response)
When you perform this operation once, it gets saved on your LTE modem. You don't need to run this code again unless you want to change the scan sequence.
Pico LTE Not Registered to Network
If the Pico LTE application or the AT+CREG?
AT command output returns "+CREG: 0,2" it indicates that your device is not registered on the network. Possible scenarios are as follows:
- If you are using the device for the first time, the network registration process may take a longer time initially, theoretically up to 5 minutes, but it is expected to register faster.
- Please check through Sixfab Connect that your device is Active and has sufficient balance.
GPS Fix Duration
GPS fix takes a few minutes usually. It depends upon the antenna and the position of the device. If the device is under open sky then time to fix gets considerably reduced.
Applications
The following are the steps to be taken to overcome possible problems when following up with practitioner tutorials.
Amazon
I'm getting "INFO: {'interval': 0, 'status': 1}" in logs, and I cannot see my message in device shadow document
It means that your endpoint address in the config.json file is corrupted or wrong. Please go to the Step 11, and provide the correct HTTP endpoint address information. Check if your file is same as the test configuration file provided above.
Pico LTE sends the message, however, the device shadow document is not changing
If the status value is "1" in the information log you receive, it means that Pico LTE is performing its task correctly. If there is no update in your Device Shadow Document in AWS, first check your topic address in your config.json file. Check that the address of this topic belongs to the publish channel named /update.
It gives me an "Certificates couldn't find in modem!" error
This error can have multiple causes. The most common of these is to upload the certificate files into your Pico LTE without changing the names of the certificate files correctly. Another reason is that the installed certificate files are not installed in the cert/ folder in the file system of your Pico LTE. Please check both these conditions and try again.
Sending GPS Data to Web Server / Telegram
+CME ERROR: 516
This error means that your modem couldn't fixed the location. Be sure that you're trying to connect GPS in outdoor, or attach a better antenna to connect easier.
+CME ERROR: 504
This error provides you an information about an ongoing process on the modem with GPS. You can ignore it and continue to your own process. It is possible to exit the ongoing job and restart your code.
HTTP Request to Web Server
+CME ERROR: 703 error when reading the response.
Please increase the sleeping time before asking the Pico LTE to read the response. It should be solved.
+CME ERROR: 712 error while sending a POST request.
This error described as wrong or empty URL setting for the host. Please check your config.json file and put the proper URL which includes "https://" and its query.
+CME ERROR: 712 error while sending a PUT request.
This error described as wrong or empty URL setting for the host. Please check your config.json file and put the proper URL which includes "https://" and its query.
Send Message to Telegram Channel
If Telegram is unable to retrieve the chatid and '/getUpdates' returns {"ok":true,"result":[]}, try sending a few random messages to the chat you created with the bot. After that, refresh the API URL page you visited in the browser.
Summary of CME ERROR Codes
Final result code +CME ERROR: indicates an error related to mobile equipment or network. The operation of +CME ERROR: final result code is similar to the regular ERROR result code: if +CME ERROR: is the result code for any of the commands in a command line, none of the following commands in the same command line is executed (neither ERROR nor OK result code shall be returned as a result of a completed command line execution).
The format of can be either numeric or verbose. This is set with AT+CMEE.
The following table lists most of general and GRPS related ERROR codes. For some GSM protocol failure cause described in GSM specifications, the corresponding ERROR codes are not included.
CME Error Code | Description |
---|---|
3 | Operation not allowed |
4 | Operation not supported |
30 | No network service |
31 | Network timeout |
103 | Memory full, cannot store entry |
106 | Undefined error |
107 | Unsupported operation |
111 | Invalid text string length |
160 | SIM not ready after reset |
171 | SIM busy |
172 | SIM wrong |
193 | SIM not ready |
194 | SIM not ready after reset |
300 | ME failure |
302 | Operation not allowed |
303 | Operation not supported |
304 | Invalid PDU mode |
305 | Invalid text mode |
310 | (U)SIM not inserted |
313 | (U)SIM failure |
314 | (U)SIM busy |
315 | (U)SIM wrong |
320 | Memory failure |
331 | No network |
332 | Network timeout |
382 | Phone failure |
500 | Unknown |
512 | (U)SIM not ready |
517 | Invalid service mode |
513 | SIM busy |
703 | HTTP(S) busy |
705 | HTTP(S) no GET/POST/PUT requests |
706 | HTTP(S) network busy |
710 | HTTP(S) network error |
711 | HTTP(S) URL error |
714 | HTTP(S) DNS error |
Please note that this table includes only some common CME error codes, and there may be additional error codes specific to the BG95 module. For a comprehensive list and detailed descriptions of all CME error codes, it is best to refer to the official documentation provided by Quectel.
Cannot Access Pico
Flash the microcontroller by dragging and dropping the UF2 file from this URL. This action will delete all files on your Pico, including MicroPython firmware. Try this approach if you're unable to access the programmable interface of the Pico through any other method.
Updated 3 months ago