Applications - Button, LED, PWM, Qwiic, NeoPixel LED
These basic components gently guide your initial experience, helping you become familiar with the device. Experiment with button functions, create simple LED displays, adjust analog signals using PWM, easily connect Qwiic sensors, and add dynamic NeoPixel LED effects to your projects. These foundational elements of Pico LTE are here to assist as you embark on your creative journey.
Blink the Onboard User LED
The USER LED on the Pico LTE turns on and off again and again. In each cycle, the led.toggle()
command changes the light from on to off and the other way around. This makes the light blink with a one-second break each time. The cycle goes on forever, making the light blink without stopping.
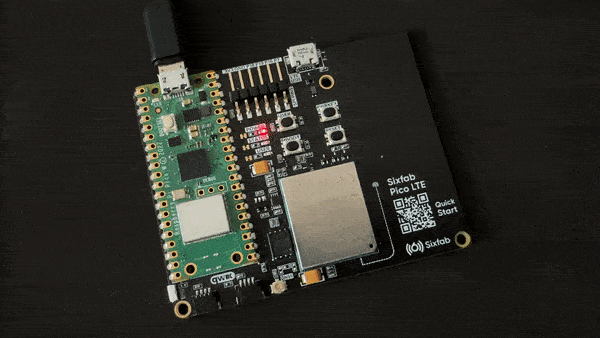
The USER LED is connected to the GP22 pin. When the pin is pulled HIGH, the LED lights up.
import machine
import utime
led = machine.Pin(22, machine.Pin.OUT)
while True:
led.toggle()
utime.sleep(1)
Button Controlled LED Toggle
In this example, we control a USER LED (connected to GP22) using a USER Button (connected to GP21). When the button is pressed, the light turns on. When the button is not pressed, the light turns off.
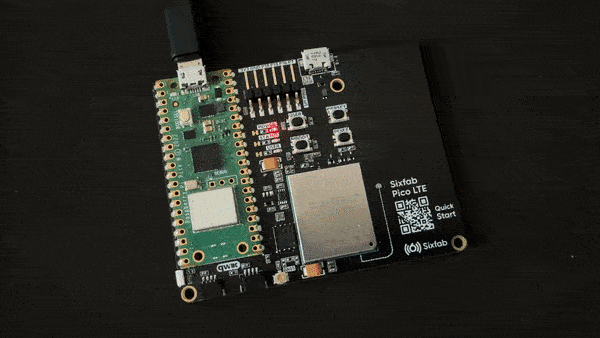
import machine
import utime
led = machine.Pin(22, machine.Pin.OUT) # We set up the pin to control the light.
button = machine.Pin(21, machine.Pin.IN, machine.Pin.PULL_DOWN) # We set up the pin for the button.
while True:
if button.value() == 0: # If the button is pressed (value is 0):
led.on() # Turn on the light.
else: # If the button is not pressed (value is 1):
led.off() # Turn off the light.
utime.sleep(0.1) # Wait for a short moment before checking again.
Inside the while True
loop, we check the button's value using button.value()
. If the button is pressed (value is 0), the LED is turned on using led.on()
, and if the button is released, the LED is turned off using led.off()
. We introduce a small delay of 0.1 seconds using utime.sleep(0.1)
to debounce the button.
Control User LED Brightness with PWM
You can control the brightness of the USER LED using Pulse Width Modulation (PWM). PWM allows you to adjust the duty cycle of a digital signal to effectively control the average voltage supplied to the LED, which in turn controls its brightness.
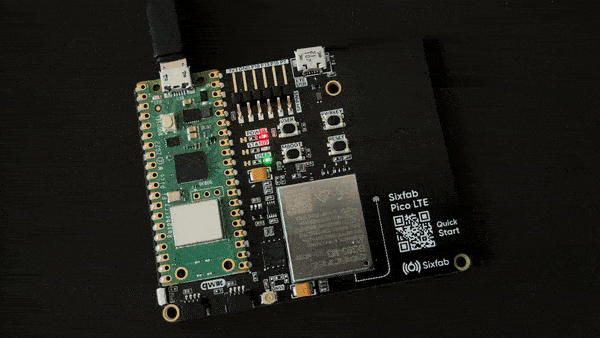
Here's how you can use PWM to control the brightness of the USER LED on the Pico LTE.
import machine
import utime
led = machine.Pin(22, machine.Pin.OUT) # Set up a pin to control the LED.
pwm_led = machine.PWM(led) # Initialize PWM (Pulse Width Modulation) for the LED.
while True:
for duty_cycle in range(0, 65535, 1024): # Increase LED brightness.
pwm_led.duty_u16(duty_cycle) # Set the LED brightness level.
utime.sleep(0.01) # Pause to observe the change.
for duty_cycle in range(64511, 0, -1024): # Decrease LED brightness.
pwm_led.duty_u16(duty_cycle) # Set the LED brightness level.
utime.sleep(0.01) # Pause to observe the change.
In this code, we create a PWM object (pwm_led
) associated with the USER LED (connected to GP22). The PWM duty cycle is adjusted using the duty_u16()
method, which takes a value between 0 and 65535 (where 0 is completely off and 65535 is fully on).
The code uses a loop to gradually increase and decrease the brightness of the LED in steps of 1024. The utime.sleep(0.01)
between each step creates a smooth transition in brightness. The LED will gradually brighten and then dim in a loop.
You can adjust the range and step values in the loops to control the rate at which the brightness changes. This will allow you to customize the behavior of the LED's brightness control.
Basic Counter Using a Button
You can make the Pico LTE count things when you press a button. This is a simple way to learn about how to use a button with the Pico LTE and make it do things when you press the button.
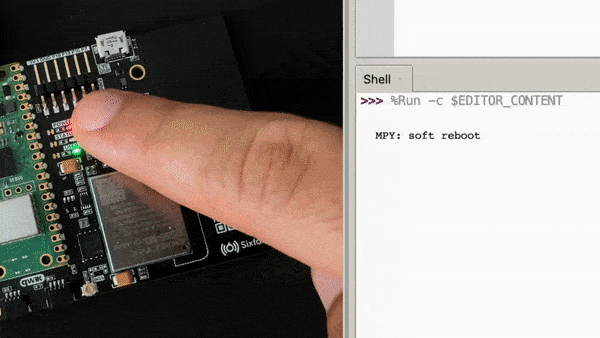
import machine
import utime
button = machine.Pin(21, machine.Pin.IN, machine.Pin.PULL_DOWN)
counter = 0
while True:
if button.value() == 0:
counter += 1
print("Counter:", counter)
while button.value() == 0:
pass # Wait for the button to be released
utime.sleep(0.1)
In this code example, we create a simple counter using a USER Button (connected to GP21) on the Pico LTE. When the button is pressed, the counter increases by one, and the updated value is printed in the terminal. The counter continues to increase as long as the button is held down. We prevent incorrect counting by introducing a short delay when the button is released.
Using this basic example, you can observe the counter increasing each time the button is pressed. You can further develop more complex projects by utilizing the counter in various ways, such as triggering an event when a specific count is reached.
Qwiic
Qwiic, a standardized I2C interface, simplifies sensor connections by allowing easy integration with various devices. The Pico LTE equipped with cellular capabilities, offers extended possibilities for IoT projects. With two Qwiic Connectors, the Pico LTE ensures effortless sensor attachment without requiring soldering, thus streamlining the prototyping process. This combination unlocks a range of benefits, enabling remote control, data collection, and fostering innovation in IoT applications.
📖 Note
Pico LTE's Qwiic I2C pins are connected to GP12 (SDA), GP13 (SCL). The two parallel-connected Qwiic sockets on the Pico LTE are connected to these pins for I2C communication.
Learn how to measure room temperature using the SparkFun Digital Temperature Sensor - TMP102 (Qwiic) with Pico LTE. Connect the sensor to the Pico easily using Qwiic connectors, no complex wiring.
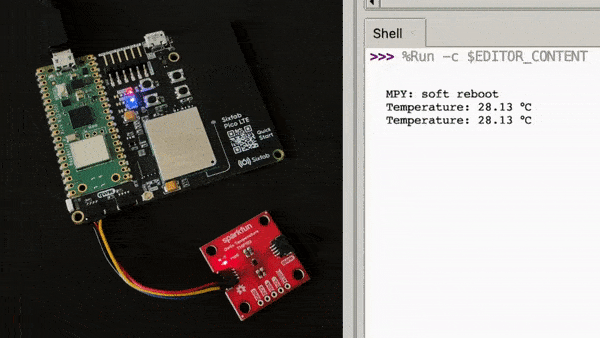
import machine
import time
# Establishing the I2C connection. '0' corresponds to the I2C driver.
# The 'SDA' and 'SCL' parameters respectively specify the SDA and SCL pins.
# The 'freq' parameter determines the I2C communication speed.
i2c = machine.I2C(0, scl=machine.Pin(13), sda=machine.Pin(12), freq=100000)
tmp102_address = 0x48 # TMP102 I2C address (default set to 0x48).
# Temperature reading function
def read_temperature():
data = i2c.readfrom(tmp102_address, 2) # Read two bytes of data
raw_temp = (data[0] << 8) | data[1] # Get the raw data combined
temperature = (raw_temp >> 4) * 0.0625 # Convert raw data to temperature value
return temperature
while True:
temperature = read_temperature()
print("Temperature: {:.2f} °C".format(temperature)) # Print the temperature value
time.sleep(1) # Slow down the loop by waiting 1 second
NeoPixel LED
A NeoPixel LED is connected to GP15 on the Pico LTE. You can learn how to control this LED easily with the examples below.
1. Color Cycling
This example moves through various colors, making a captivating color-changing result.
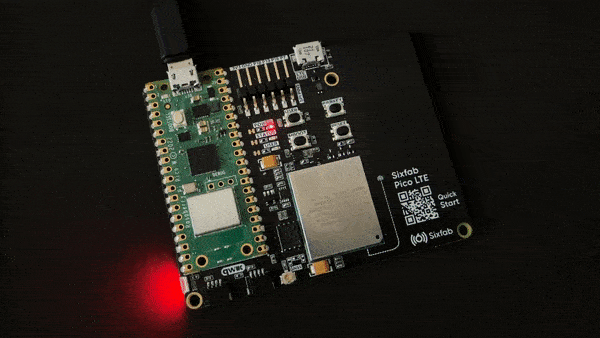
import machine
import neopixel
import utime
NUM_LEDS = 8
pin = machine.Pin(15)
np = neopixel.NeoPixel(pin, NUM_LEDS)
def color_cycle(wait):
for i in range(NUM_LEDS):
np[i] = (255, 0, 0) # Set LED color to red
np.write()
utime.sleep_ms(wait) # Wait for a short duration
for i in range(NUM_LEDS):
np[i] = (0, 255, 0) # Set LED color to green
np.write()
utime.sleep_ms(wait) # Wait for a short duration
for i in range(NUM_LEDS):
np[i] = (0, 0, 255) # Set LED color to blue
np.write()
utime.sleep_ms(wait) # Wait for a short duration
while True:
color_cycle(100) # Call the color cycle function with a delay of 100 milliseconds
This code makes NeoPixel LEDs change colors in a cycle, going through red, green, and blue. This creates synchronized and equally timed color shifts.
2. Rainbow Effect
This example creates a smooth rainbow effect across the NeoPixel LED strip.
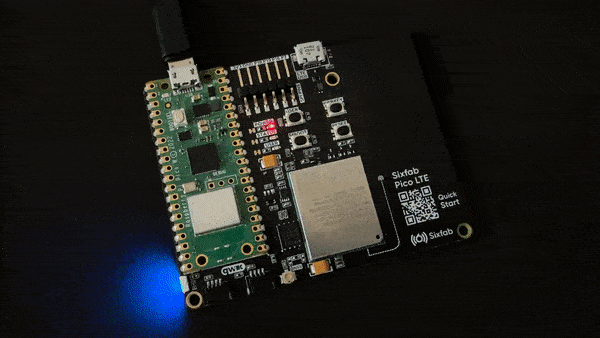
import machine
import neopixel
import utime
NUM_LEDS = 8
pin = machine.Pin(15)
np = neopixel.NeoPixel(pin, NUM_LEDS)
# Function for rainbow effect
def rainbow_cycle(wait):
for j in range(255):
for i in range(NUM_LEDS):
rc_index = (i * 256 // NUM_LEDS) + j
np[i] = wheel(rc_index & 255) # Set LED color using wheel function
np.write()
utime.sleep_ms(wait)
def wheel(pos):
if pos < 85:
return (255 - pos * 3, pos * 3, 0) # Red to Green transition
elif pos < 170:
pos -= 85
return (0, 255 - pos * 3, pos * 3) # Green to Blue transition
else:
pos -= 170
return (pos * 3, 0, 255 - pos * 3) # Blue to Red transition
while True:
rainbow_cycle(20) # Call the rainbow effect function with a delay of 20 milliseconds
The rainbow_cycle
function uses the wheel function to generate RGB color values for each LED, resulting in a captivating visual display.
Please remember, these examples and ideas are just the beginning. You can explore more complex projects by combining various sensors, displays, and actuators with the Raspberry Pi Pico to create unique and engaging applications.
Updated 2 months ago